Beginners Guide: Building a Mentor Chatbot using Pro Config on MyShell.ai
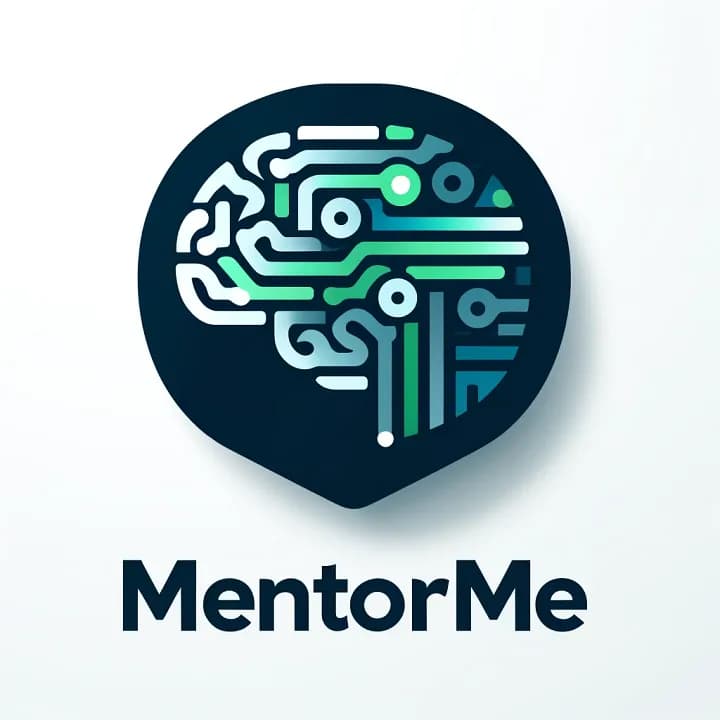
Introduction
MentorMe is an AI application designed to assist users in overcoming challenges in software development, including solving complex coding problems, debugging development issues, and addressing mental blocks. This guide will walk you through how to build this app step-by-step using MyShell's ProConfig feature.
Before we begin
MentorMe is comprised of four main states:
- Home State: The starting point where users choose their path.
- Leetcode State: Assists with solving Leetcode problems.
- Development State: Provides help for debugging code.
- Mental Blocks State: Offers support for overcoming mental hurdles.
Step-by-Step Setup
Step 1: Create the chatbot
- Log in to your MyShell.ai account.
- Navigate to the dashboard and select "Workshop".
- Select "Create a Bot".
- Provide an Avatar Photo and Name for your Bot.
- Select "No" for NSFW.
- Select the learning tag under the Tag drop down.
- Add your Description and Intro Message for your Bot.
Example Description:
Have access to a software engineering mentor at any time! MentorMe is here to guide you through the toughest leet code problems, development hurdles and mindset blocks. If you are stuck on a problem, need help getting started on a project or feel mentally drained or unconfident on where to begin- MentorMe is here to help! Start chatting today.
Example Intro Message:
Welcome to MentorMe! 🌟 Your 24/7 software engineering mentor is here to guide you through the toughest LeetCode challenges, development obstacles, and mindset hurdles. We understand that the journey to becoming a software engineer can be daunting, but with MentorMe, it just got a whole lot easier! Whether you're stuck on a problem, need a kickstart on a project, or are feeling mentally drained and unsure of where to begin, we're here to help. Start chatting with us today and take your coding skills to the next level! Let's conquer those coding challenges together! 💻✨
Step 2: Create the Home State
Root Level
{
"type": "automata",
"id": "coding_mentor",
"initial": "home_state",
"states": {
// Holds the different states of the application
...
}
}
"type": "automata" Specifies this JSON object's type.
"id": "coding_mentor" Provides a unique identifier for the application.
"initial": "home_state" Indicates the entry point of the application when it is first launched.
States Object
"states": {
"home_state": {
...
}
// Additional states will be defined here
}
"states" A container for all the individual states the application can be in. Each state has its own set of properties and behaviors.
Home State
"home_state": {
"type": "text", // This state involves text interaction
"render": { // This is what will be shown to the user
...
},
"transitions": { // Handles the transitions from this state to others
...
},
//Leetcode State Here
}
"type": "text" Indicates that this state involves text-based interaction, such as reading and responding to text.
"render" Specifies what will be displayed to the user in this state.
Render Object
"render": {
"text": "Allow me to help you along your journey of becoming a **Senior Developer**! 💻✨ \n **Leetcode Challenges**, **Bugs** or **Mental Blocks** won't stand in your way anymore. **Excited to get started**? \n\n **How can I help**?",
"buttons":[
{
"content": "Leetcode problem",
"description": "I'm here to help you understand and solve any LeetCode coding challenges.",
"on_click": "leetcode"
},
{
"content": "Development",
"description": "I'm here to help you with any development bug you may encounter.",
"on_click": "development"
},
{
"content": "Mental Block",
"description": "I'm here to help you with any mental blocks you face along the way.",
"on_click": "mental_blocks"
}
]
}
"text" Provides the introductory text that will be displayed to the user. This can include markdown for formatting.
"buttons" An array of buttons that provide interactive elements for the user.
Transitions Object
"transitions": {
"leetcode": "leetcode_state",
"development": "development_state",
"mental_blocks": "mental_blocks_state"
}
Each key is an action that results from the user interacting with the corresponding button, and the value is the name of the state to which the application should transition.
Step 3: Create the LeetCode State
Inputs Object
"leetcode_state": {
"inputs": {
"problem": {
"type": "text",
"description": "Please provide the details of the LeetCode problem.",
"user_input": true
},
"language": {
"type": "text",
"choices": [
"C++", "Java", "Python", "C", "C#", "Javascript",
"Typescript", "PHP", "Swift", "Kotlin", "Dart", "Go",
"Ruby", "Scala", "Rust", "Racket"
],
"description": "Select your programming language",
"user_input": true
}
}
}
"type": "text" Specifies the expected input format. For both 'problem' and 'language', the input should be text.
"description" Provides guidance on what information the user should provide or select.
"user_input": true Indicates that these fields are mandatory for user interaction.
"choices" Lists the programming languages available for selection, ensuring the user knows their options.
Tasks Object
"tasks": [
{
"name": "leetcode_solver",
"module_type": "AnyWidgetModule",
"module_config": {
"widget_id": "1744214345505574912",
"content": "Solve the leetcode problem using this programming language {{language}} and explain why this is the best way to solve the problem. Here is the leetcode problem: {{problem}}. Ensure that it passes all test cases, provide best solution. Do not use built-in libraries",
"output_name": "solution"
}
}
]
"name" Unique identifier for the task within the system.
"module_type" Indicates the type of module used, allowing for flexibility in task processing.
"widget_id" A unique identifier for the specific widget used, linking the task configuration to a concrete implementation.
"content" Instructions for the task, dynamically filled with user inputs.
"output_name" Defines where the task's result is stored, making it accessible for further processing or display.
Render Object
"render": {
"text": "{{solution}}",
"buttons": [
{
"content": "Try Again",
"description": "let's do it again",
"on_click": "regenerate"
},
{
"content": "Thank You",
"description": "I am satisfied with your solution!",
"on_click": "goodbye"
}
]
}
"text" Displays the solution generated by the task.
"buttons" Provides interactive elements for the user, allowing them to either retry the task or conclude the session.
"on_click" Defines actions triggered by button clicks, linked to state transitions for a dynamic user experience.
Transitions Object
"transitions": {
"regenerate": "leetcode_state",
"goodbye": "home_state"
}
Specifies the next states the application should transition to based on user interactions, ensuring a fluid and responsive user journey.
Step 4: Create the Development State
The Development State follows a similar structure to the LeetCode State, with some specific differences in the inputs and tasks.
Inputs Object
"development_state": {
"inputs": {
"error": {
"type": "text",
"description": "Please provide any bugs or errors you've encountered while working on development.",
"user_input": true
}
}
}
Captures error or bug reports from users, essential for debugging and enhancing the application.
Tasks Object
"tasks": [
{
"name": "found",
"module_type": "AnyWidgetModule",
"module_config": {
"widget_id": "1744214345505574912",
"content": "provide the best possible solution of this error [{{error}}], solution should be details oriented. Provide detailed explanation for developers understanding.",
"output_name": "found_solution"
}
}
]
"name" Identifies the task within this specific state; here, it's called "found" because the goal of this task is to find a solution.
"widget_id" Same as used in previous states.
"content" Instructional prompt that outlines the task for the widget, which involves providing a detailed solution to a reported error.
"output_name" The variable name where the result of this task is stored.
Render Object
"render": {
"text": "{{found_solution}}",
"buttons": [
{
"content": "Try Again",
"description": "let's do it again",
"on_click": "regenerate"
},
{
"content": "Thank You",
"description": "I am satisfied with your solution!",
"on_click": "goodbye"
}
]
}
"text" Outputs the text result from the found_solution
variable.
"buttons" Similar to the previous section, these buttons offer options to either redo the task or complete the interaction.
Transitions Object
"transitions": {
"regenerate": "development_state",
"goodbye": "home_state"
}
Defines where the system transitions based on user actions, maintaining consistency across states with functional and logical flow.
Step 5: Create the Mental Blocks State
The Mental Blocks State has a slightly different structure, designed to handle more open-ended conversations about mental challenges in coding.
Inputs Object
"mental_blocks_state": {
"inputs": {
"user_message": {
"type": "IM",
"user_input": true
}
}
}
"type": "IM" Indicates an instant messaging format for user interaction, designed for real-time communication.
Tasks Object
"tasks": [
{
"name": "generate_reply",
"module_type": "AnyWidgetModule",
"module_config": {
"widget_id": "1744214024104448000",
"system_prompt": "You are a teacher, teaching how to relieve mental blocks while coding and maintain general good mental health to software developers. Ask for what the user needs help with before providing any answer. Then answer the {{user_message}}. When answering remember to always ask if the user has any follow-up questions.",
"user_prompt": "{{user_message}}",
"output_name": "reply"
}
},
{
"name": "generate_voice",
"module_type": "AnyWidgetModule",
"module_config": {
"widget_id": "1743159010695057408",
"content": "{{reply}}",
"output_name": "reply_voice"
}
}
]
"generate_reply" A task designed to process the user's message and generate a relevant response.
"generate_voice" Converts the text response into speech, enhancing accessibility and interaction.
"system_prompt" and "user_prompt" These fields guide the AI's response, ensuring it is contextually relevant to the user's query.
"content" (under generate_voice) Specifies the text to be converted into speech.
Render Object
"render": {
"text": "{{reply}}",
"audio": "{{reply_voice}}",
"buttons":[{
"content": "Thank You",
"description": "I am satisfied with your help!",
"on_click": "goodbye"
}]
}
"audio" Plays the voice response, adding a dynamic and interactive element to the user experience.
Transitions Object
"transitions": {
"CHAT": "mental_blocks_state",
"goodbye": "home_state"
}
"CHAT" Allows the user to remain in the "mental_blocks_state" for continuous interaction, reflecting a conversational flow.
Conclusion
Great job completing this tutorial and building your very own mentor app using Pro Config! Be sure to test each path through the application to ensure that transitions are smooth and that outputs are correct.
Let's do a quick review of what we've covered:
- Initial set up.
- How to create the structure of the JSON config.
- Creating the
home_state
. - Input definitions for the
leetcode_state
,development_state
, andmental_blocks_state
, detailing the expected data type, user interaction requirement, and purpose of each input field. - Task configurations in all states, including task names, module types, widget IDs, content instructions, and output names for storing results.
- Render configurations that specify how results (text or audio) are displayed and the actions associated with buttons for user interaction.
- Transition mappings that define how the system moves from one state to another based on user actions.
Thank you for reading! You can find the MentorMe chatbot here.
About the Author
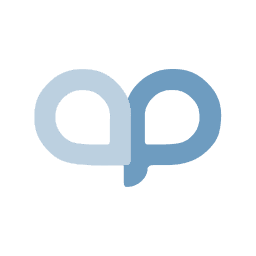
Angel Pichardo
Developer Relations Engineer specializing in AI and developer education. With extensive experience in creating technical content and building developer communities, Angel is passionate about making complex technologies accessible to all developers.